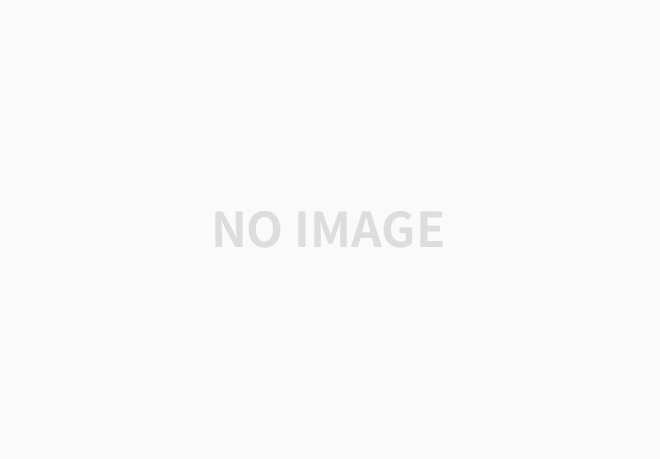
Last episode
Last episode we discussed how writing programs in native machine code, and having to contend with so many low level details, was a huge impediment to writing complex programs.
To abstract away many of these low-level details, Programming Languages were developed that let programmers concentrate on solving a problem with computation, and less on nitty gritty hardware details.
Today's episode
So today, we’re going to continue that discussion, and introduce some fundamental building blocks that almost all programming languages provide.
statement & syntax
Just like spoken languages, programming languages have statements.
These are individual complete thoughts, like “I want tea” or “it is raining”.
By using different words, we can change the meaning;
for example, “I want tea” to “I want unicorns”.
But we can’t change “I want tea” to “I want raining” - that doesn’t make grammatical sense.
The set of rules that govern the structure and composition of statements in a language is called syntax.
The English language has syntax, and so do all programming languages.
assignment statement
“A equals 5” is a programming language statement. In this case, the statement says a variable named A has the number 5 stored in it.
This is called an assignment statement because we're assigning a value to a variable.
To express more complex things, we need a series of statements, like “A is 5, B is ten, C equals A plus B”
This program tells the computer to set variable ‘A’ equal to 5, variable ‘B’ to 10, and finally to add ‘A’ and ‘B’ together, and put that result, which is 15, into variable C.
Note that we can call variables whatever we want.
Instead of A, B and C, it could be apples, pears, and fruits.
The computer doesn’t care, as long as variables are uniquely named.
But it’s probably best practice to name them things that make sense in case someone else is trying to understand your code.
program
A program, which is a list of instructions, is a bit like a recipe: boil water, add noodles, wait 10 minutes, drain and enjoy.
In the same way, the program starts at the first statement and runs down one at a time until it hits the end.
So far, we’ve added two numbers together. Boring.
Let’s make a video game instead!
Of course, it’s way too early to think about coding an entire game, so instead, we’ll use our example to write little snippets of code that cover some programming fundamentals.
Imagine we’re building an old-school arcade game where Grace Hopper has to capture bugs before they get into the Harvard Mark 1 and crash the computer!
On every level, the number of bugs increases.
Grace has to catch them before they wear out any relays in the machine. Fortunately, she has a few extra relays for repairs.
initialize
To get started, we’ll need to keep track of a bunch of values that are important for game-play, like what level the player is on, the score, the number of bugs remaining, as well as the number of spare relays in Grace’s inventory.
So, we must “initialize” our variables, that is, set their initial value: “level equals 1, score equals 0, bugs equals 5, spare relays equals 4, and player name equals “Andre”.
Control Flow Statements
To create an interactive game, we need to control the flow of the program beyond just running from top to bottom.
To do this, we use Control Flow Statements.
If Statements (Conditional Statements)
There are several types, but If Statements are the most common. You can think of them as “If X is true, then do Y”.
An English language example is: “If I am tired, then get tea”
So if “I am tired” is a true statement, then I will go get tea If “I am tired” is false, then I will not go get tea.
An IF statement is like a fork in the road.
Which path you take is conditional on whether the expression is true or false -- so these expressions are called Conditional Statements.
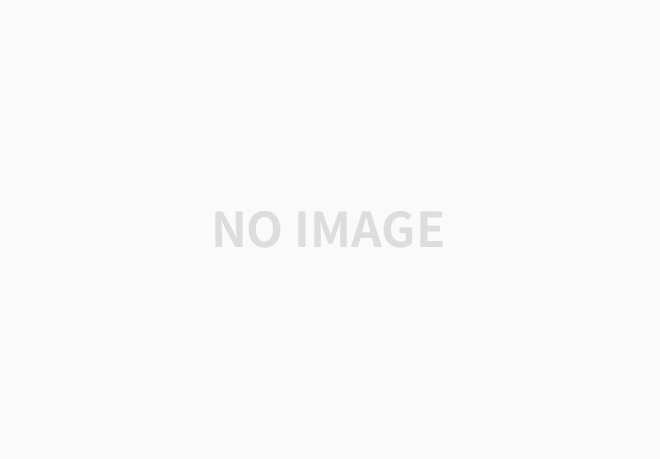
Here are some examples of if-then-else statements from some popular programming languages -- you can see the syntax varies a little, but the underlying structure is roughly the same.
If-statements are executed once, a conditional path is chosen, and the program moves on.
Conditional loop
To repeat some statements many times, we need to create a conditional loop.
One way is a while statement, also called a while loop.
As you might have guessed, this loops a piece of code “while” a condition is true.
For Loop
There’s also the common For Loop.
Instead of being a condition-controlled loop that can repeat forever until the condition is false, a FOR loop is count-controlled; it repeats a specific number of times.
A new level of abstraction
What we want is a way to package up our exponent code so we can use it, get the result, and not have to see all the internal complexity.
We’re once again moving up a new level of abstraction!
Functions (subroutines, methods)
To compartmentalize and hide complexity, programming languages can package pieces of code into named functions, also called methods or subroutines in different programming languages.
These functions can then be used by any other part of that program just by calling its name.
When we call a single line of code, like this the complexity is hidden.
We don’t see all the internal loops and variables, we just see the result come back as if by magic. But it’s not magic, it’s the power of abstraction!
If you understand this example, then you understand the power of functions, and the entire essence of modern programming.
It’s not feasible to write, for example, a web browser as one gigantically long list of statements.
It would be millions of lines long and impossible to comprehend!
So instead, software consists of thousands of smaller functions, each responsible for different features.
In modern programming, it’s uncommon to see functions longer than around 100 lines of code, because by then, there’s probably something that should be pulled out and made into its own function.
Modularizing programs into functions not only allows a single programmer to write an entire app, but also allows teams of people to work efficiently on even bigger programs.
Different programmers can work on different functions, and if everyone makes sure their code works correctly, then when everything is put together, the whole program should work too!
Libraries
And in the real world, programmers aren’t wasting time writing things like exponents.
Modern programming languages come with huge bundles of pre-written functions, called Libraries.
These are written by expert coders, made efficient and rigorously tested, and then given to everyone.
There are libraries for almost everything, including networking, graphics, and sound -- topics we’ll discuss in future episodes.
이번 에피소드는 프로그래밍의 기초인 Statement와 Function에 대해서 배울 수 있었다.
자바스크립트를 배우면서 계속해서 구현해야 하는 부분이었어서, 다른 에피소드와는 달리 생소하지는 않았고 영어로 개념을 다시 다질 수 있어서 좋았다.
또 영상에서 직접 게임을 만들면서 for, function등을 만들어 나가는데, 나중에 게임을 어떻게 만들어 나가면 될지에 대한 아이디어를 준다.
'Computer Science > Crash Course' 카테고리의 다른 글
Software Engineering: Crash Course Computer Science #16 (0) | 2021.10.18 |
---|---|
Alan Turing: Crash Course Computer Science #15 (0) | 2021.10.16 |
The First Programming Languages: Crash Course Computer Science #11 (0) | 2021.10.13 |
Early Programming: Crash Course Computer Science #10 (0) | 2021.10.12 |
Advanced CPU Designs: Crash Course Computer Science #9 (0) | 2021.10.11 |